Code quality is an essential pillar that determines not only the efficiency and functionality of the end product, but also its ability to adapt and evolve to new requirements and technological challenges. In this context, the SOLID principles emerge as a beacon of best practices in object-oriented design and programming, providing a theoretical and practical framework for building more robust, maintainable, and scalable applications.
Introduced by Robert C. Martin, affectionately known as “Uncle Bob,” these five fundamental principles provide developers with tools to address some of the most common problems in software development, such as tight coupling, low cohesion, and difficulty in testing.
In this article, we will explore each of these principles in detail, unraveling their meaning and applicability through concrete examples, and highlighting how their integration can transform the software development process into a more elegant and sustainable practice.
Single Responsibility Principle (SRP)
The Single Responsibility Principle (SRP) is one of the foundations of the SOLID principles and states that each class in a software system should have a single reason for change. This means that each class should focus on a single functionality or responsibility within the system, thus avoiding overloading classes with multiple behaviors or responsibilities.
The importance of SRP lies in its ability to facilitate clean, modular software design. By ensuring that each class has a single responsibility, code becomes more readable, maintainable, and amenable to effective unit testing. In addition, this principle reduces system complexity, simplifies the development process, and avoids unexpected side effects when modifying or extending code.
The resulting benefits can be summarized as improved maintainability, easier test writing, greater reusability, and less coupling by reducing dependencies between classes.
Implementing the SRP
To adhere to the SRP, it is critical to perform a detailed analysis of the system requirements and functionalities to identify and separate the various responsibilities. Each class should be designed to encapsulate a single functionality or aspect of the system. If a class is found to handle more than one responsibility, consideration should be given to refactoring the class into smaller classes, each focused on a single task or function.
Common traps and anti-patterns
As we just discussed, sometimes classes are overloaded with additional responsibilities. For example, a Book class might contain not only properties and methods related to a book, but also logic for storing that book in a database. This mixing of domain logic and persistence logic is a common anti-pattern that violates the SRP.
To comply with SRP, you can separate the persistence logic into another class, ensuring that each class has only one reason to change. This approach makes the code not only more maintainable, but also more scalable.
Practical example
Next, we will discuss an example in Python applied to an employee management system in a company. Initially, we have an Employee class that manages both the employee’s personal information and salary calculation operations.
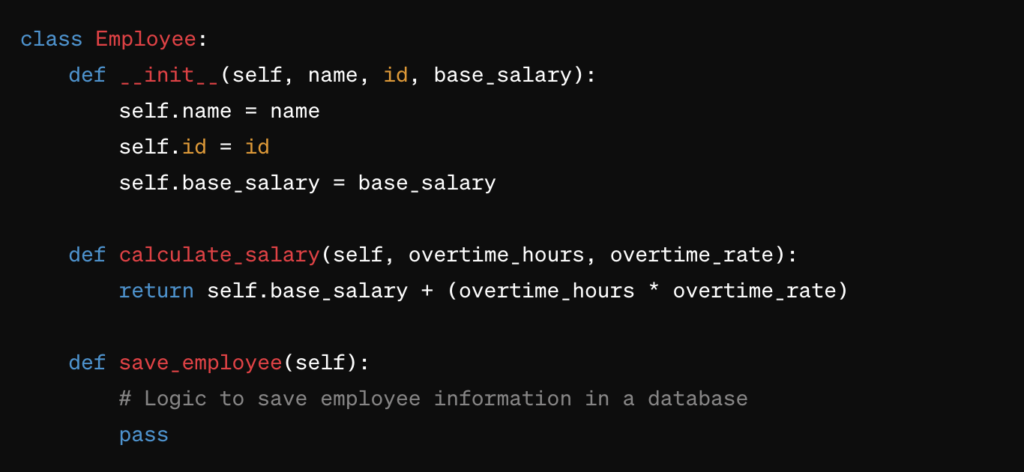
In this design, the Employee class has multiple responsibilities: maintaining employee information and managing the employee’s salary, as well as persisting this data. This violates the SRP, since there is more than one reason to change this class.
To adhere to the SRP, we can split this class into two: one that exclusively handles the employee’s personal and salary information, and one that handles the persistence of the employee’s data.
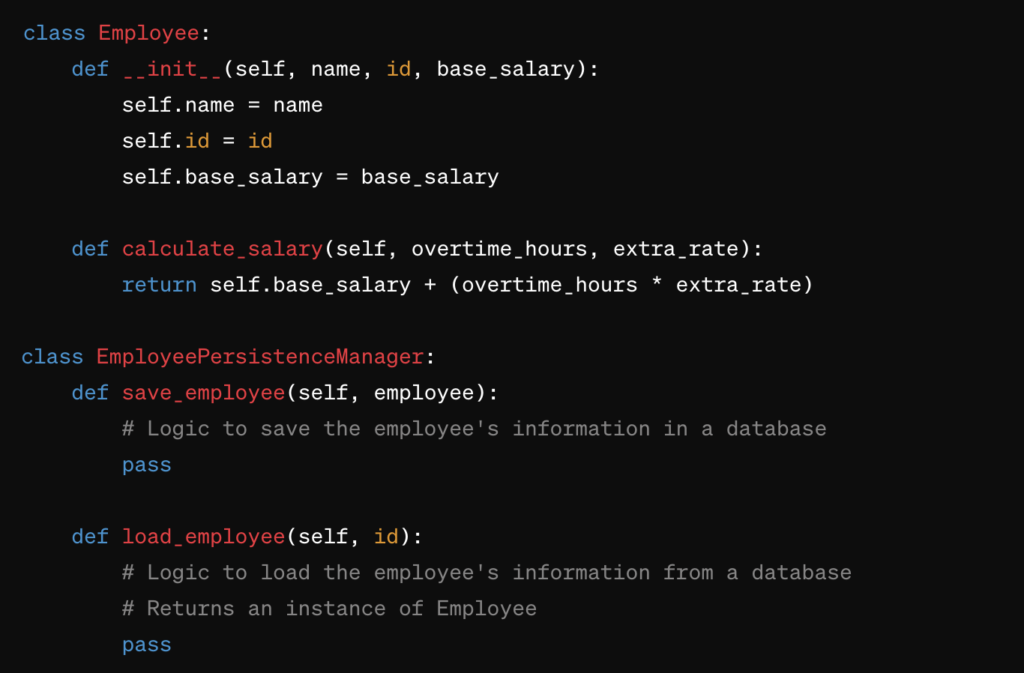
In this approach, Employee focuses on the properties and behaviours directly related to the employee, while EmployeePersistenceManager handles all employee-related database interactions. This separation of responsibilities makes the code more modular, easier to maintain and understand.
Open/Closed Principle (OCP)
The Open/Closed Principle (OCP) states that software entities (classes, modules, functions, etc.) should be open for extension but closed for modification. This means that a software component should be extensible without the need to modify the existing source code.
In this sense, the OCP allows the software to grow and change with new or changing requirements, minimizing the risk of introducing bugs into already tested and functional code. This reduces the cost and effort associated with implementing new functionality or adapting to different contexts or requirements.
OCP implementation
OCP implementation is often achieved through the use of abstractions (such as interfaces or abstract classes) and composition over inheritance. This allows behaviours to be extended by modifying how an object is composed (through dependency injection, for example) rather than changing existing behaviour.
Practical example
Next, we will discuss an example in Python applied to a payment processing system where you need to handle different types of payments (credit card, PayPal, cryptocurrencies, etc.). Without the OCP, you could find yourself constantly adding new conditions and methods to a payment processing class every time you need to support a new payment method, which violates the principle of being “closed for modification”.
A solution that follows the OCP could be to define an IPayment interface with a processPayment() method.
Then, for each payment method, you create a class that implements IPayment (such as CreditCardPayment, PayPalPayment, CryptocurrencyPayment) as shown below:

This design allows new payment methods to be added to the system by simply creating new classes that implement IPago, without the need to modify the existing code that processes payments. This complies with the OCP by being closed for modifications but open for extensions.
Liskov Substitution Principle (LSP)
The Liskov Substitution Principle (LSP), formulated by Barbara Liskov in 1987, is a fundamental concept in object-oriented design. This principle states that if a class S is a subtype of a class T, then objects of type T in a program can be replaced by objects of type S (i.e., objects of the subclass) without changing any of the desirable properties of the program (correctness, task it performs, etc.).
The LSP is critical to the design of robust and maintainable systems because it promotes code interoperability and reusability. By adhering to the LSP, developers can extend and modify systems with confidence, knowing that new subtypes will not introduce bugs or unexpected behavior.
LSP Implementation
To adhere to the LSP, it is essential to ensure that subclasses do not change the behavior of superclasses in ways that may surprise the user of the code. This includes respecting superclass invariants, adhering to method contracts (including parameter and return value requirements), and not introducing new exceptions that cannot be handled.
Practical example
Next, we show how an interface-based solution that distinguishes between birds that can fly and birds that cannot fly could be introduced using Python:
First, we define a general interface for Bird and another one for birds that can fly, FlyingBird.
Then, we implement specific classes for different types of birds, making sure that only birds that can fly implement the BirdFlying interface as seen below:

In this design, Bird can eat and fly, so it implements both Bird and FlyingBird. On the other hand, Penguin, which can eat but not fly, implements only Bird. This satisfies the LSP, since we can replace any Bird with either a Bird or a Penguin without changing the expected behaviour with respect to the ability to eat. However, we can only expect a Flying Bird to fly.
This approach ensures that our classes comply with the LSP because it prevents subclasses (such as Penguin) from being forced to implement methods (such as Flying) that they cannot use, maintains consistency, and avoids incorrect or unexpected behaviour.
Interface Segregation Principle (ISP)
The Interface Segregation Principle (ISP) addresses how interfaces in software should be structured to promote a clean, modular design. In essence, the ISP suggests that “clients should not be forced to rely on interfaces that they do not use”.
Implementation of the ISP is critical to avoid interface “bloat,” where an interface is loaded with too many methods that are not relevant to all of its consumers. This leads to the creation of dependent implementations of parts of the interface that they don’t need, which can result in code that is difficult to maintain and understand, as well as increasing the risk of runtime errors.
ISP implementation
To comply with the ISP, interfaces must be specific to the needs of your customers, rather than generic. This means breaking down large, comprehensive interfaces into smaller, more specific sets that are relevant to their respective customers. This promotes a cleaner design, where implementations only need to know and rely on the interfaces they actually use.
Practical example
Imagine a management system for a library that includes operations such as printing loan receipts, sending email notifications, and saving loan records. Initially, you could have a large LibraryServices interface with methods for each of these operations. However, not all clients of this interface will need all of these operations. For example, a class that only handles printing receipts should not need to implement methods for sending emails or saving records.
To apply the ISP to the library management system, we will split the large interface LibraryServices into smaller, specialized interfaces, such as IReceiptService, INotificationService, and IRecordService by defining them according to their functionalities. Each customer would then implement only the interfaces relevant to their needs, thus promoting a cleaner, more modular design.
Next, we implement specific classes that only need to implement the interfaces relevant to their responsibilities:

In this design, each service focuses on a single responsibility and implements only the interface that corresponds to that responsibility. For example, ReceiptService is only concerned with printing receipts, so it only implements IReceiptService. This means that if a part of the system only needs to handle receipt printing, it can rely solely on IReceiptService without worrying about the other functionality.
This approach not only complies with the ISP, but also makes the code easier to maintain and more extensible, since adding new functionality or modifying existing functionality has minimal impact on the other parts of the system.
Dependency Inversion Principle (DIP)
The Dependency Inversion Principle (DIP) focuses on how dependencies are structured within a software system, promoting a structure that facilitates maintainability and flexibility. DIP states that:
- High-level modules should not depend on low-level modules. Both should depend on abstractions.
- Abstractions should not depend on details. Details must depend on abstractions.
The application of DIP is crucial to avoid rigid coupling between software modules, allowing for a system that is easier to modify, extend and test. By relying on abstractions rather than concrete implementations, high-level modules are not directly tied to the details of low-level modules, which promotes a more modular and reusable design.
DIP implementation
DIP implementation often involves the use of interfaces or abstract classes to define the abstractions that form the contract between different modules of a system. High-level modules that contain business logic or policies define their dependencies in terms of these interfaces, while low-level modules that implement specific details such as database operations or network communications implement these interfaces.
Practical example
For this case, we will use as an example an e-commerce application with a high-level module that manages customer orders and a low-level module that handles access to the product database.
Without DIP, the order management module could be directly dependent on the specific implementation of the database access module, making it difficult, for example, to switch to a new database or data source.
Applying DIP, we would introduce an abstraction (e.g., an IProductRepository interface) that the order management module would use to interact with the products.
Next, we implement the database access module that manages the products, ProductRepository, which implements the IProductRepository interface. This could represent, for example, an access to a relational database:

Now, we create the order management module, OrderManager, which depends on the IProductRepository abstraction instead of a concrete implementation, following the DIP:
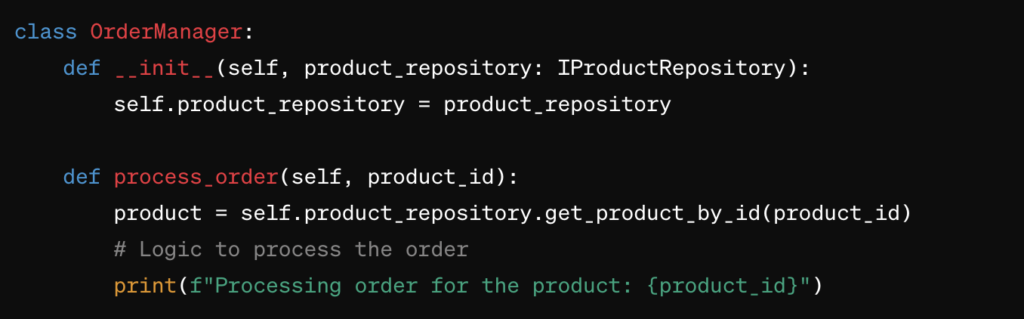
Finally, in the configuration part of our application or at the entry point, we inject the specific dependency of the ProductRepository in OrderManager:

This design allows you to easily change the implementation of the product repository without modifying the OrderManager, for example, if you decide to switch from a relational database to a NoSQL one. You just need to create a new class that implements ProductRepository for the new database and change the instance passed to OrderManager. This demonstrates how DIP facilitates a flexible and easily maintainable design.
Conclusion
Adopting the SOLID Principles is a significant investment in the quality and sustainability of software development. Through the exploration and practical application of each principle, developers can gain a greater understanding and appreciation for software design that not only effectively meets current needs, but also facilitates future adaptation and growth.
As we have seen throughout this article, the importance of these principles lies in their ability to lead to cleaner, decoupled, and easily extensible code, paving the way for systems that can fluidly evolve in the face of changing business needs and technological advances.
Therefore, incorporating SOLID principles into the development process is not just a best practice, but a philosophy that promotes excellence in software design and ensures that every line of code contributes to building the robust, maintainable, and scalable applications that are ultimately at the heart of technological innovation.
Want to learn more about programming? Don’t miss these resources!
- Guide to finding the ideal programming language
- How to choose the best code repository for your project
- Software development best practices: Keys to excellence
- Best practices in software development: Integrating OOP
At Block&Capital, we strive to create an environment where growth and success are accessible to all. If you’re ready to take your career to the next level, we encourage you to join us.
Last posts