Software development is a discipline that combines creativity, logic and continuous adaptation to new technologies and methodologies. Among these, Object-Oriented Programming (OOP) stands out as a fundamental pillar to build robust, maintainable and scalable software.
In this article we will delve into how the conscious integration of this concept contributes to good practices in software development.
Object Oriented Programming (OOP)
OOP is a programming paradigm that uses objects and classes as fundamental elements for the construction of software. It also promotes a more organized and reusable code structure, facilitating the management of complex projects. Therefore, adopting OOP leads to a more modular code, where changes in one part of the system have a minimal impact on the others, improving maintainability and scalability.
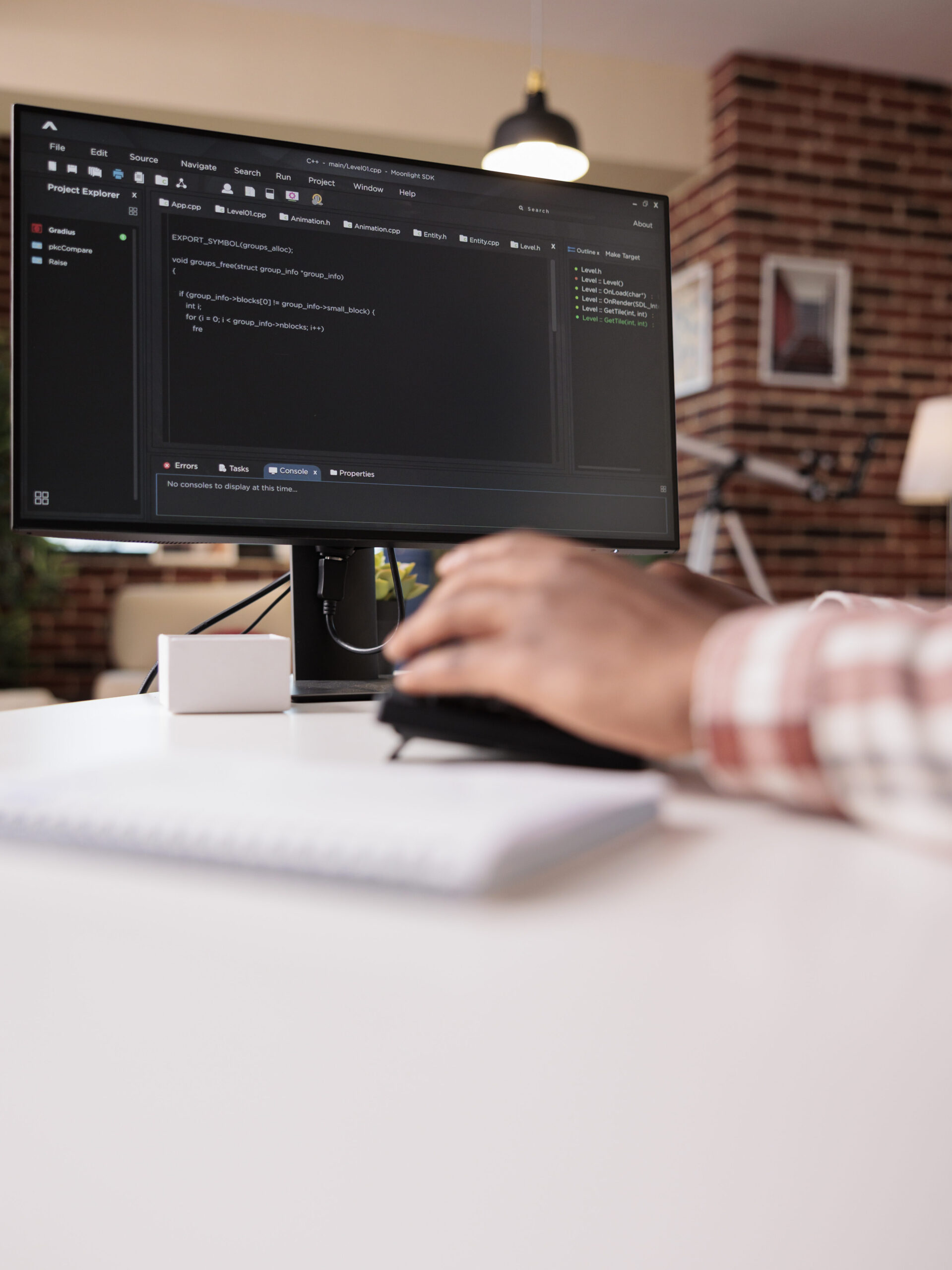
The 4 pillars of Object-Oriented Programming are:
- Abstraction: Facilitates understanding and complexity management by focusing on the essential aspects of objects, separating them from specific or less relevant details.
- Encapsulation: Internal details are hidden and only the necessary characteristics and behaviors of an object are exposed to the outside, ensuring that the internal state is protected from unauthorized access and modification.
- Inheritance: Allows the creation of new classes (subclasses) from existing classes (superclasses), avoiding code duplication.
- Polymorphism: Allows objects of different classes to respond differently to the same operations.
We will now take a closer look at each of these pillars:
Abstraction
In the context of OOP, abstraction is used to create simplified models or representations of real or conceptual systems. This allows developers to define classes that represent abstract object types with common properties and behaviors, but without specifying the full details that make up those objects.
For example, consider a vehicle management system for a car rental company. In this system, you can have an abstraction “Vehicle” that encapsulates the common characteristics and behaviors of all vehicles, as shown below:
- Abstraction: Vehicle.
- Essential aspects (attributes): make, model, color, fuel type, license plate.
- Behaviors (methods): start(), stop(), accelerate(), brake().
This abstraction ignores the specific details that differentiate one type of vehicle from another, allowing all vehicles to be treated as a single vehicle.
Encapsulation
Encapsulation refers to the practice of hiding the internal details of a class implementation and exposing only the necessary features and behaviors of an object to the outside. This concept is essential for achieving a high degree of security and safety in object interactions, allowing developers to limit access to the internal states of objects and control how information is accessed or modified.
In essence, encapsulation allows a class to group its related variables (attributes) and functions (methods), keeping some internal details hidden and exposing only what is necessary for external use. This is achieved through the use of access modifiers such as private, protected and public, which define the visibility of the members of a class.
For example, in a BankAccount class, you could have private attributes such as balance and public methods such as deposit() and withdraw(). The balance attribute is not directly accessible from outside the class, thus protecting the integrity of the data. It can only be interacted with through the deposit() and withdraw() methods, which implement the necessary rules to ensure that the balance is never negative.
A BankAccount class that manages the account balance would be defined this way in Python:
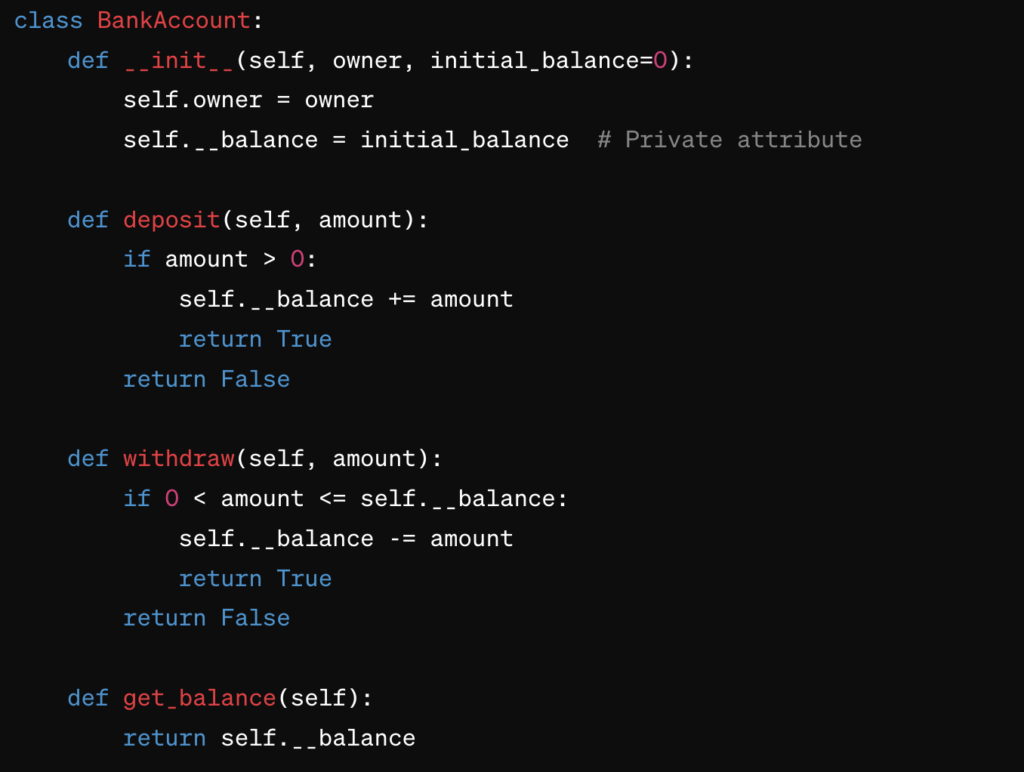
In this example, the account balance is encapsulated and protected from direct access and modification. The deposit() and withdraw() methods provide a controlled interface to change the balance, while get_balance() allows accessing the balance without modifying it.
Inheritance
Inheritance is a key concept in object-oriented programming (OOP) that allows a class (called a subclass or derived class) to inherit attributes and methods from another class (called a superclass or base class). This inheritance mechanism facilitates code reuse and the creation of hierarchical relationships between classes, allowing developers to build complex object structures in an efficient and organized manner.
Thus, inheritance promotes the principle of “no code repetition” by allowing new classes to adopt existing features of other classes without having to rewrite them. In addition, it provides a way to implement polymorphism and specialization through method overwriting, where subclasses can modify or expand inherited behaviors as needed.
For example, consider a class hierarchy where you have a superclass Vehicle with general properties such as make, model, and methods such as start() or stop(). From this base class, you could derive more specific subclasses such as Car, Motorcycle or Truck, each with additional attributes and behaviors that are unique to that type of vehicle, but also sharing the common attributes and behaviors defined in the Vehicle superclass.
This example, where we extend the Vehicle abstraction to create subclasses such as Car, is defined in Python this way:
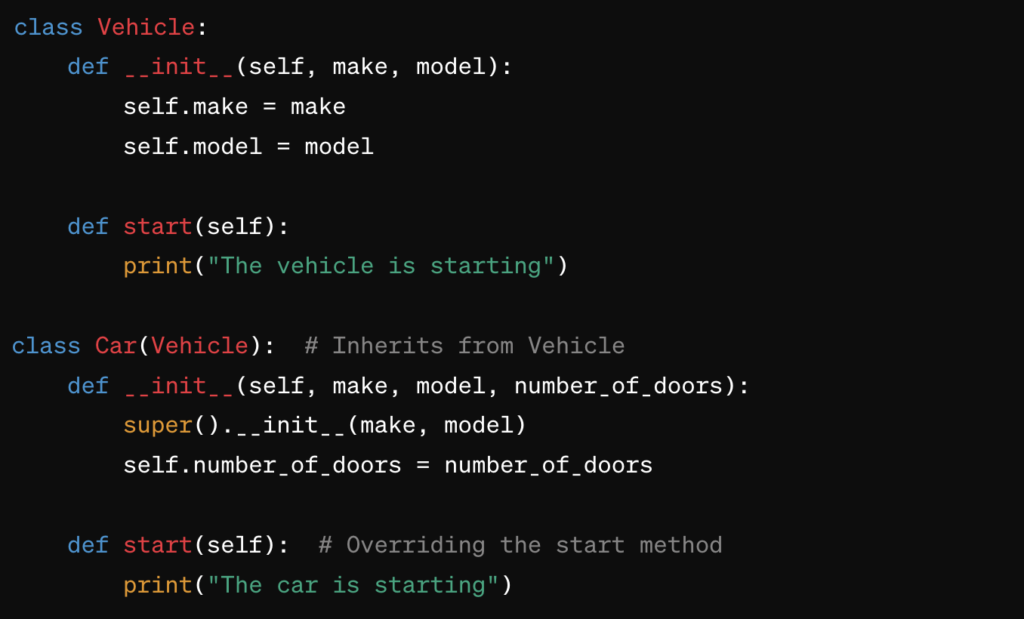
As you can see, here Car inherits from Vehicle and adds a new attribute (number_of_doors). In addition, you can override methods (such as start()) to behave in a specific way.
Polymorphism
When we talk about polymorphism, we refer to the ability of an object to assume multiple forms. In the context of OOP, this means that an object of a superclass can be represented as an object of a subclass, and methods that have the same name can behave differently depending on the object that invokes them. This allows programmers to use a common interface for different underlying types of objects, which increases the flexibility and reusability of the code.
There are mainly two types of polymorphism in OOP: run-time polymorphism (also known as dynamic polymorphism) and compile-time polymorphism (static polymorphism).
Runtime polymorphism is achieved by using inheritance and method overwriting (for example, when a derived class redefines a method of its base class), while compile-time polymorphism is achieved by overloading methods and operators.
Let’s look at a practical example in Python where polymorphism is used to interact with different types of vehicles:
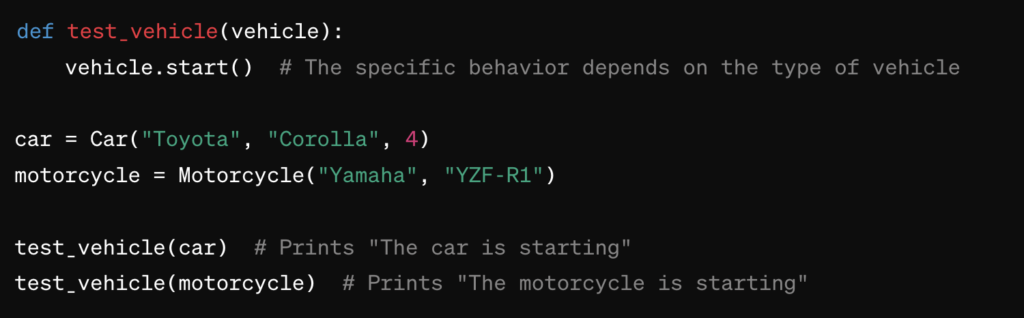
Here, test_vehicle is a function that can accept any object that is a subtype of Vehicle. Depending on the type of object passed to the function, the corresponding start() method will be invoked, demonstrating polymorphism.
Conclusion
Object-Oriented Programming (OOP) has proven to be an invaluable approach to software development, offering a structure that not only reflects the real world in a more natural way but also facilitates the creation, maintenance and scalability of complex applications. Through its four pillars, OOP enables developers to design robust, flexible and reusable systems.
By adopting OOP, development teams can significantly improve the quality and lifespan of their software systems. This approach not only improves code organization and makes it easier to understand and debug, but also makes the process of adapting to new requirements simpler and less error-prone.
With its focus on modularity, reusability and abstraction, OOP remains an essential methodology for building high-quality software while maintaining the agility needed to adapt to a constantly evolving technological world.
Want to learn more about programming? Don’t miss these resources!
- Guide to find the ideal programming language
- How to choose the best code repository for your project
- Software development best practices: Keys to excellence
At Block&Capital, specialists in tech recruitment, we strive to create an environment where growth and success are within everyone’s reach. If you’re ready to take your career to the next level, we encourage you to join us.
Last posts